If you ever want to know the breakdown of domains for a list of emails without the rigmarole of having to import them into a database first, then here is a simple but handy JavaScript tool to do just that (which means you don’t need any special software to use it – any web browser should do the trick).
Step 1: Create your input form
First create your form which will have a textarea to input the list of emails, an input for the number of domains you want to output, a run button and a reset button.
<form name="form1">
<table>
<tr>
<td><h3>Input Emails:</h3>
<fieldset>
<textarea rows="30" cols="40" name = "TextAreaInput"></textarea>
</fieldset>
<fieldset>
<strong>No. Domains to Count:</strong> <input type = "text" name = "NoDomains" value = "20" size = "5"/><br><br>
</fieldset>
<fieldset>
<input type="button" Value="Display Domains" onclick="run(this.form);"/>
</fieldset>
<fieldset>
<input type="button" value="Reset" onClick="this.form.reset();cleardiv()" />
</fieldset></td>
<td style="padding-left: 50px; padding-right: 50px"></td>
<td bgcolor=#2E2E2E></td><td style="padding-left: 50px; padding-right: 50px"></td>
<td><br><br><div id='pastetable'></div></td></tr>
</table>
</form>
The final <td> element to the table will be a placeholder for the counts that are generated using the run() function which is a <div></div> area which can allow the html code of the domain counts table to be inserted.
Step 2: Create your functions
This tool will require several functions.
- Turn your text input into an array which can then be processed.
function textareaToArray(t)
{
return t.value.split(/[\n\r]+/);
}
This function will take a string and create an array line by line, separating elements out using returns and newlines before returning the final array.
- Clear the table results during the reset
function cleardiv()
{
var target = document.getElementById("pastetable");
target.innerHTML = '';
}
Since the reset() function only refers to the form we created, we need to add another function to clear the results of the domain counts after they have been displayed in our placeholder area. This function will simply replace the area within the <div></div> of our table with nothing, thus removing the table.
- Improve the formatting of the counts table
function FormatNumber(x)
{
return x.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
}
JavaScript doesn’t really have its own formatting function for numbers, so this confusing looking function will simply add a comma in a number for every three digits it finds before a character that is not a number using regexp.
- Generate the domain counts
Leaving the best until last, this function will work out the counts for each domain and select the top counts per the user input from the form, before turning it into HTML code to be sent to our placeholder within the main HTML:
function run(form1)
{
var counter = 0;
// Pull the form data, the list of emails go into the variable “Emails” and are changed into an array
// The number of domain are put into “NoDomains”
var Emails = textareaToArray(document.form1.TextAreaInput);
var NoDomains = document.form1.NoDomains.value;
// For each email replace the prefix and @ sign with nothing leaving just the domain behind
while (counter<Emails.length)
{
Emails[counter] = Emails[counter].replace(/.*@/, "");
counter = counter +1;
}
// Create an array of JSON using Domain and Total which will be 1 for later summing
var EmailsSort = [];
var counter2 = 0;
while (counter2<Emails.length)
{
EmailsSort[counter2]={Domain:Emails[counter2],Total:1};
counter2 = counter2+1;
}
// Set the variable “result” to an array of distinct domains with all their totals added up and then sort by Total
var result = EmailsSort.reduce(function(res, obj) {
if (!(obj.Domain in res))
res.__array.push(res[obj.Domain] = obj);
else {
res[obj.Domain].Total += obj.Total;
}
return res;
}, {__array:[]}).__array
.sort(function(a,b) { return b.Total - a.Total; });
// Generate the HTML code looping round every element of “result” for as many times as the user requested outputting the Domain name and the formatted number for Total
var counter3 = 0;
var tableoutput1 = '<table border = 1 cellpadding="8" width="400"><tr><td width="250"><strong>Domain</strong></td><td><strong>Count</strong></td></tr>';
var currenttotal = 0;
while (counter3<NoDomains)
{
if(counter3<result.length){
currenttotal = result[counter3].Total;
tableoutput1 = tableoutput1+ '<tr><td>'+result[counter3].Domain + '</td><td>' + FormatNumber(result[counter3].Total) +'</td></tr>' ;
}
counter3 = counter3+1;
}
tableoutput1 = tableoutput1+'</table>';
var target = document.getElementById("pastetable");
target.innerHTML = tableoutput1;
}
Step 3: Run it
It’s as simple as pasting in some emails and pressing a button:
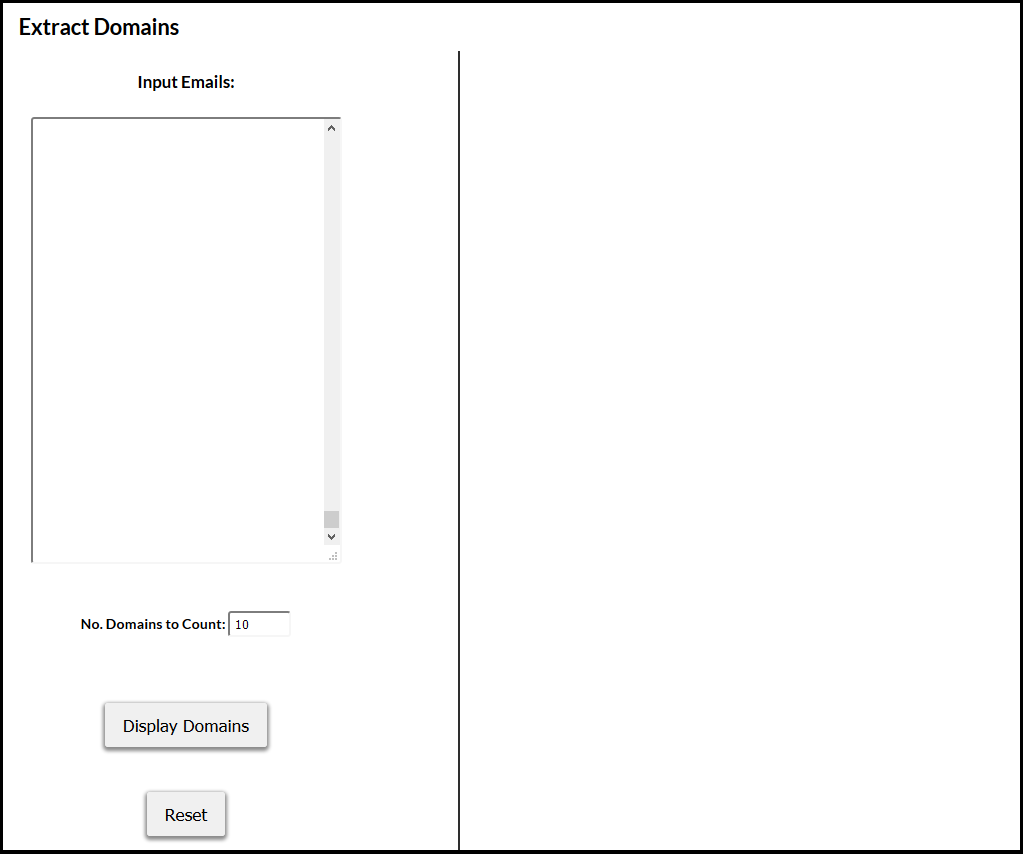
Then your results should just appear, as if by magic:
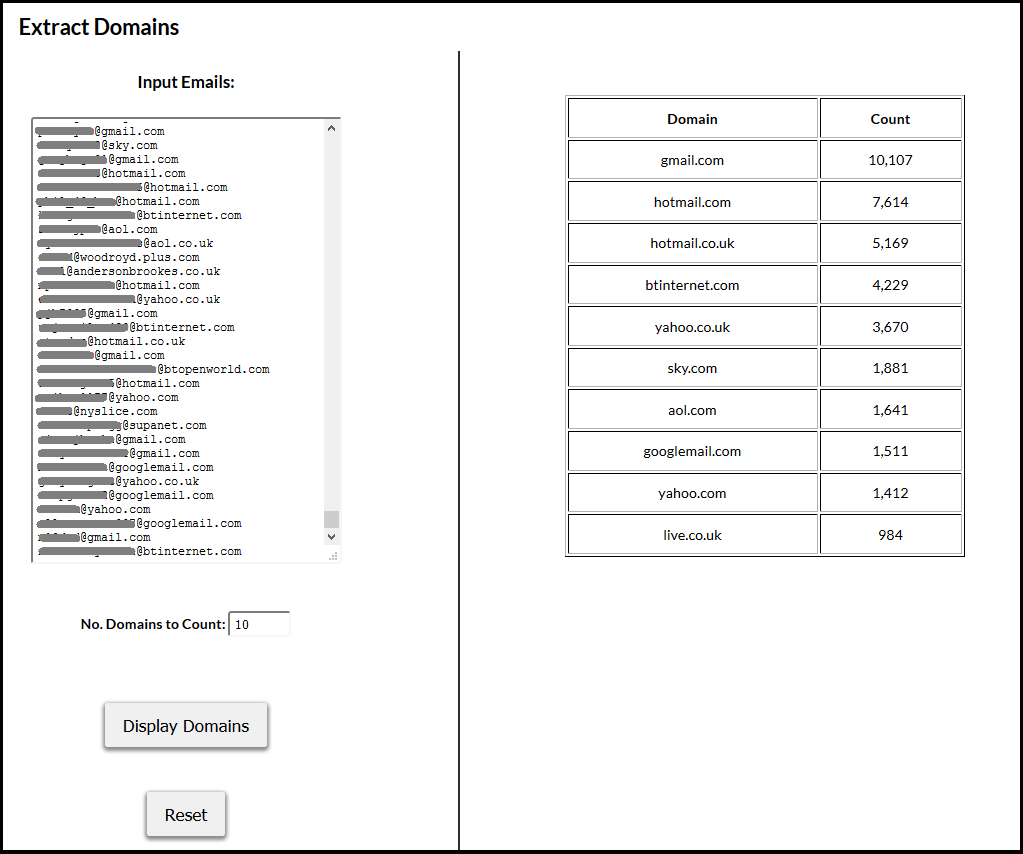
I’ve pushed the boat out and tried with over 1 million emails in one go to see if it could handle it, and whilst the browser hung during the pasting of the emails, the actual computation took mere seconds so all in all, it’s a pretty robust tool. You can find a complete copy of the html here: